Line data Source code
1 : //! # LIEF
2 : //!
3 : //! 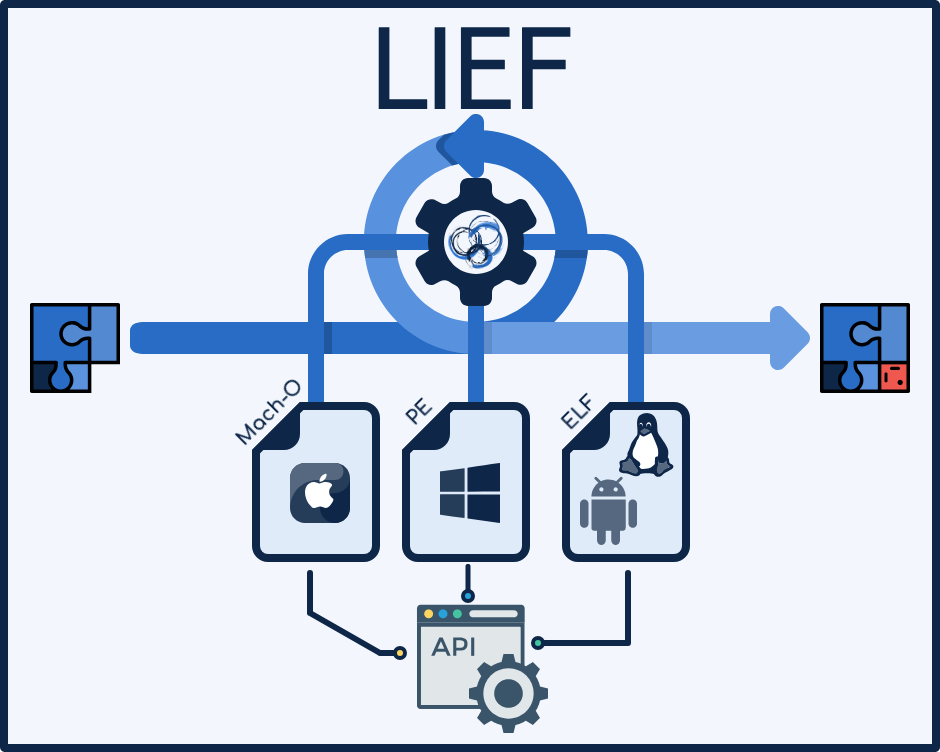
4 : //!
5 : //! This package provides Rust bindings for [LIEF](https://lief.re). It exposes most of the
6 : //! LIEF API to **read** these formats:
7 : //! - ELF
8 : //! - PE
9 : //! - Mach-O
10 : //!
11 : //! The bindings require at least Rust version **1.74.0** with the 2021 edition and support:
12 : //! - Windows x86-64 (support `/MT` and `/MD` linking)
13 : //! - Linux x86-64/aarch64/musl (Ubuntu 19.10, Almalinux 8, Debian 10, Fedora 29)
14 : //! - macOS (`x86-64` and `aarch64` with at least OSX Big Sur: 11.0)
15 : //! - iOS (`aarch64`)
16 : //!
17 : //! ## Getting Started
18 : //!
19 : //! ```toml
20 : //! [package]
21 : //! name = "my-awesome-project"
22 : //! version = "0.0.1"
23 : //! edition = "2021"
24 : //!
25 : //! [dependencies]
26 : //! # For nightly
27 : //! lief = { git = "https://github.com/lief-project/LIEF", branch = "main" }
28 : //! # For releases
29 : //! lief = 0.15.0
30 : //! ```
31 : //!
32 : //! ```rust
33 : //! fn main() {
34 : //! let path = std::env::args().last().unwrap();
35 : //! let mut file = std::fs::File::open(path).expect("Can't open the file");
36 : //! match lief::Binary::from(&mut file) {
37 : //! Some(lief::Binary::ELF(elf)) => {
38 : //! // Process ELF file
39 : //! },
40 : //! Some(lief::Binary::PE(pe)) => {
41 : //! // Process PE file
42 : //! },
43 : //! Some(lief::Binary::MachO(macho)) => {
44 : //! // Process Mach-O file (including FatMachO)
45 : //! },
46 : //! None => {
47 : //! // Parsing error
48 : //! }
49 : //! }
50 : //! return;
51 : //! }
52 : //! ```
53 : //!
54 : //! Note that the [`generic`] module implements the different traits shared by different structure
55 : //! of executable formats (symbols, relocations, ...)
56 : //!
57 : //! ## Additional Information
58 : //!
59 : //! For more details about the install procedure and the configuration, please check:
60 : //! <https://lief.re/doc/latest/api/rust/index.html>
61 :
62 : #![doc(html_no_source)]
63 :
64 : pub mod elf;
65 :
66 : /// Executable formats generic traits (LIEF's abstract layer)
67 : pub mod generic;
68 :
69 : pub mod macho;
70 :
71 : pub mod pe;
72 :
73 : pub mod pdb;
74 :
75 : pub mod dwarf;
76 :
77 : pub mod objc;
78 :
79 : pub mod dsc;
80 :
81 : pub mod debug_info;
82 :
83 : pub mod assembly;
84 : mod range;
85 :
86 : /// Module for LIEF's error
87 : pub mod error;
88 :
89 : pub mod logging;
90 :
91 : mod binary;
92 : mod common;
93 :
94 : mod debug_location;
95 :
96 : #[doc(inline)]
97 : pub use binary::Binary;
98 :
99 : #[doc(inline)]
100 : pub use generic::Relocation;
101 :
102 : #[doc(inline)]
103 : pub use error::Error;
104 :
105 : #[doc(inline)]
106 : pub use debug_info::DebugInfo;
107 :
108 : #[doc(inline)]
109 : pub use range::Range;
110 :
111 : #[doc(inline)]
112 : pub use debug_location::DebugLocation;
113 :
114 : /// Whether it is an extended version of LIEF
115 74930 : pub fn is_extended() -> bool {
116 74930 : lief_ffi::is_extended()
117 74930 : }
118 :
119 : /// Return details about the extended version
120 0 : pub fn extended_version_info() -> String {
121 0 : lief_ffi::extended_version_info().to_string()
122 0 : }
123 :
124 : /// Try to demangle the given input.
125 : ///
126 : /// This function requires the extended version of LIEF
127 0 : pub fn demangle(mangled: &str) -> Result<String, Error> {
128 0 : to_conv_result!(
129 : lief_ffi::demangle,
130 : *mangled,
131 0 : |e: cxx::UniquePtr<cxx::String>| { e.to_string() }
132 : );
133 0 : }
|